Text 1: Rendering and Editing
Milestones to Deleting Words with a Pixel Buffer.
As of: 2023-06-27
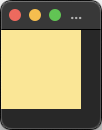
- The buffer does not have to match the screen dimensions.
- macOS screens have twice the pixel density of typical monitors. Moving the window across monitors will require a complete redraw.
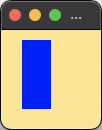
- This implementation visits every pixel in the buffer. A better approach would be to only visit the rect's bounding box.
- Proper hinting, ignoring hinting, hinting data without LERP.
- Drawing beyond the buffer's x-axis will simply wrap to the left side of the screen, similar to the Asteroids game.
- There is no need to create an actual bitmap/atlas.
- The font is CozetteVector.
- Specifying the line height is required.
- The font is VictorMono-Regular.
- Text rendering is far more complex than I initial imagined.
- Capturing input from the keyboard is trivial.
- The library implementers allow for the use of
scancode
or the VirtualKeyCode
. I can only assume that one of them will become the primary once I look into supporting alternative keyboard layouts and languages.
- This implementation currently uses the most direct approach, where it clears the entire buffer before drawing to the screen. At some point, I'll need to figure out how to just mark the area as dirty and only redraw the enclosed content.
- Repeat key presses need to be handled raw.
- Non characters such as
\u{8}
(backspace), \r | \u{3}
(return), and \n
(new line) can be ignored for now.
- Only the Escape key (to quit) required the capture of a "Key Up" event.
- The cursor position should always start at 0 unless the area is activated by the mouse.
- The width is determined by the glyphs
advance
attribute, not the width provided by the metrics to support fonts that aren't monospaced.
- The height should use the entire line height, not just the glyph's descent. (watch "g").
- The application will crash if the cursor's position is at the end of the text when the delete key is pressed. (nice).
- The content is drawn layer by layer. (background, glyphs, cursor block, cursor glyph).
- The cursor's glyph color can be set to anything.
- If the cursor's position exceeds the length of the text, I use the average glyph width according to the font's metrics.
- The cursor's height should probably use the underline offset as well, but I'll circle back once I need to.
- Using both CTRL and ALT as the key to jump for now. I'll circle back once it becomes a problem.
- Easy kill! Simply find the next or previous space and jump to it. If the space doesn't exist, go to the end or the start of the string.
This looks like the best stopping point. To get here, I had to treat the TextField like a black box entity with an API. To move the cursor left, I simply call the struct's cursor_left()
or cursor_left_by_word()
functions. The field itself then becomes the argument for the viewport's draw_text_field()
. This implemendation doesn't support anything you'd expect from a rich text editor like colors and weight.
Text 2: Building a Text Editor
Thank you.